Learning Pygame by writing a basic program
In this article, I will show you how to write a basic program that uses movement. Pygame is an open-source library that allows users to create video games.
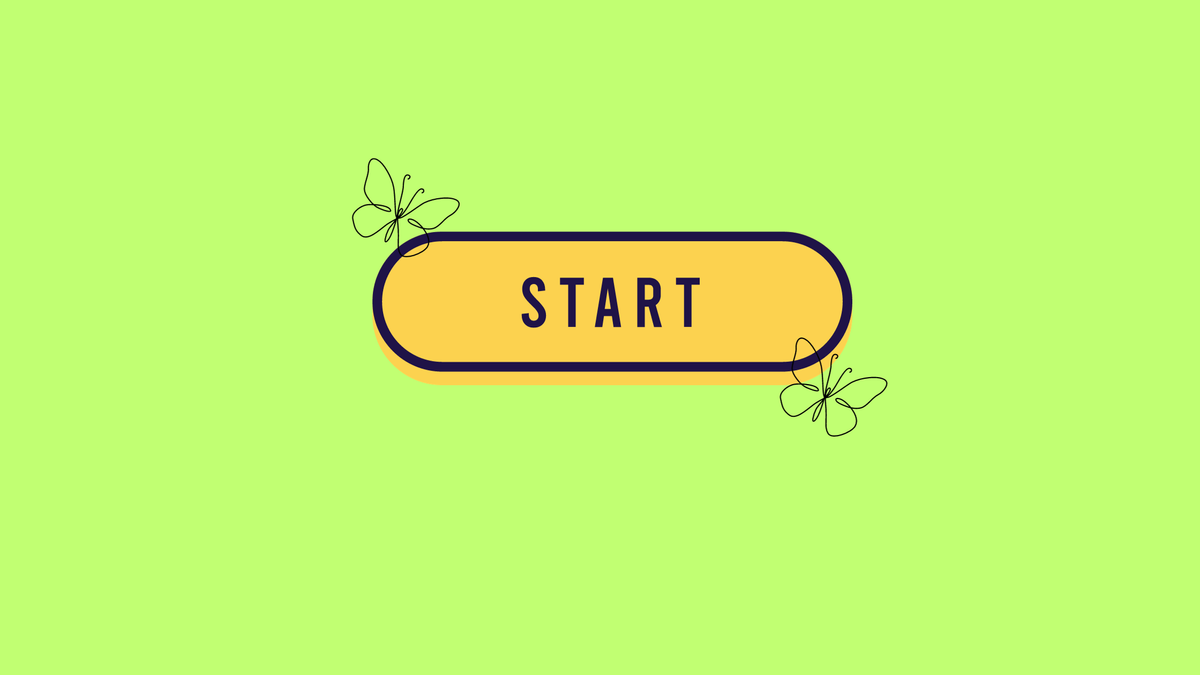
I started programming with Python again for a new side project. What better way to practice than by creating a small video game? In this article, I will show you how to write a basic program that uses movement.
Pygame is an open-source library that allows users to create video games. It is easy to install and use for beginners even though it is not used often in professional game development.
Download
Go to the official Pygame website install and download. You can either install it through pip or source code.
Getting started
Pygame offers a generous quickstart guide. Pygame is a fun way to learn Python and game development. For example, making a snake game is a good way to showcase Pygame's features.
Creating assets
For the asset, I created and used in this demo. I used Kenney's creature-mixer on itch.io. After creating the creature, I saved it and placed it in a directory called assets
. This folder will be used later in the example below.
Creating the test game
In the beginning, import the Python libraries.
import pygame
import os
Next, we are going to initialize the screen and Pygame setup.
pygame.init()
We are going to set the height and width of the screen and the sprite that was created.
WIDTH = 1280
HEIGHT = 720
SPRITE_WIDTH = 680
SPRITE_HEIGHT = 620
The name of the screen is going to be My test game program.
clock = pygame.time.Clock()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('My test game program')
Creating the game object class
The game object class will make up the bulk of the program. Here is where the player controls will be defined.
Let's start by creating a function to move the creature and load the image.
def __init__(self, image, height, speed):
self.speed = speed
self.image = image
self.pos = image.get_rect().move(0, height)
def move(self, up=False, down=False, left=False, right=False):
if right:
self.pos.right += self.speed
if left:
self.pos.right -= self.speed
if down:
self.pos.top += self.speed
if up:
self.pos.top -= self.speed
def load_image(name):
path = os.path.join(main_dir, "assets", name)
return pygame.image.load(path).convert()
Next, we have our main function where all the magic is going to happen. We are going to start by making the background pink. You can change the color to blue, red, green, etc. On the screen, we are going to display some text.
background = pygame.Surface(screen.get_size())
background = background.convert()
background.fill("pink")
# Display some text
font = pygame.font.Font(None, 36)
text = font.render("Hello, Welcome to my game!", 1, (10, 10, 10))
textpos = text.get_rect()
textpos.centerx = background.get_rect().centerx
background.blit(text, textpos)
What if we want to add some music? Pygame allows audio files can be played. Load in the audio you want. To loop it use play
.
pygame.mixer.music.load('your audio file')
pygame.mixer.music.play(3)
Load the image and put everything on the screen.
player = load_image("creature.gif")
screen.blit(background, (0, 0))
An object is going to be created for the player. The player can move the creature using the w, a, s,d keys and the up, down, left, right arrow keys on the keyboard.
#create player object
p = GameObject(player, 10, 3)
# Event loop
while True:
keys = pygame.key.get_pressed()
if keys[pygame.K_UP]:
p.move(up=True)
if keys[pygame.K_DOWN]:
p.move(down=True)
if keys[pygame.K_LEFT]:
p.move(left=True)
if keys[pygame.K_RIGHT]:
p.move(right=True)
if keys[pygame.K_w]:
p.move(up=True)
if keys[pygame.K_s]:
p.move(down=True)
if keys[pygame.K_a]:
p.move(left=True)
if keys[pygame.K_d]:
p.move(right=True)
screen.blit(background, (0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
return
screen.blit(p.image, p.pos)
clock.tick(60) # limits FPS to 60
pygame.display.update()
pygame.time.delay(100)
Lastly, the main function is called.
if __name__ == "__main__":
main()
pygame.quit()
Here is the full example
import pygame
import os
# Initialise screen and pygame setup
pygame.init()
# Height and Width of screen
WIDTH = 1280
HEIGHT = 720
# Height and width of the sprite
SPRITE_WIDTH = 680
SPRITE_HEIGHT = 620
clock = pygame.time.Clock()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('My test game program')
main_dir = os.path.split(os.path.abspath(__file__))[0]
# our game object class
class GameObject:
def __init__(self, image, height, speed):
self.speed = speed
self.image = image
self.pos = image.get_rect().move(0, height)
# move the object.
def move(self, up=False, down=False, left=False, right=False):
if right:
self.pos.right += self.speed
if left:
self.pos.right -= self.speed
if down:
self.pos.top += self.speed
if up:
self.pos.top -= self.speed
# quick function to load an image
def load_image(name):
path = os.path.join(main_dir, "assets", name)
return pygame.image.load(path).convert()
def main():
# Fill background
background = pygame.Surface(screen.get_size())
background = background.convert()
background.fill("pink")
# Display some text
font = pygame.font.Font(None, 36)
text = font.render("Hello, Welcome to my game!", 1, (10, 10, 10))
textpos = text.get_rect()
textpos.centerx = background.get_rect().centerx
background.blit(text, textpos)
#play music
pygame.mixer.music.load('your audio file')
pygame.mixer.music.play(3) # Plays audio four times, not three times
#load images
player = load_image("creature.gif")
#put everything on screen
screen.blit(background, (0, 0))
#create player object
p = GameObject(player, 10, 3)
# Event loop
while True:
# Get all keys currently pressed, and move when an arrow key is held.
keys = pygame.key.get_pressed()
if keys[pygame.K_UP]:
p.move(up=True)
if keys[pygame.K_DOWN]:
p.move(down=True)
if keys[pygame.K_LEFT]:
p.move(left=True)
if keys[pygame.K_RIGHT]:
p.move(right=True)
if keys[pygame.K_w]:
p.move(up=True)
if keys[pygame.K_s]:
p.move(down=True)
if keys[pygame.K_a]:
p.move(left=True)
if keys[pygame.K_d]:
p.move(right=True)
screen.blit(background, (0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
return
screen.blit(p.image, p.pos)
clock.tick(60)
pygame.display.update()
pygame.time.delay(100)
if __name__ == "__main__":
main()
pygame.quit()